miniosl¶
miniosl, facade as a Python module.
Many functions are imported from minioslcc
implemented in C++20.
- class miniosl.UI(init: str | BaseState | MiniRecord = '', *, prefer_text=None, default_format='usi')[source]¶
general interface for shogi state (
State
, board position + pieces in hand), enhanced with move history (MiniRecord
) and other utilities- Parameters:
init – initial contents handled by
load_record()
prefer_text – preference of state visualization
default_format – preference in __str__
make the initial state in shogi
>>> shogi = miniosl.UI()
or read csa/usi file (local or web)
>>> url = 'http://live4.computer-shogi.org/wcsc33/kifu/WCSC33+F7_1-900-5F+dlshogi+Ryfamate+20230505161013.csa' >>> shogi = miniosl.UI(url) >>> _ = shogi.go(10) >>> print(shogi.to_csa(), end='') P1-KY-KE * -KI * * -GI-KE-KY P2 * -HI-GI * -OU * -KI-KA * P3-FU * -FU-FU-FU-FU-FU-FU-FU P4 * * * * * * * * * P5 * -FU * * * * * +FU * P6+FU * * * * * * * * P7 * +FU+FU+FU+FU+FU+FU * +FU P8 * +KA+KI * * * +GI+HI * P9+KY+KE+GI * +OU+KI * +KE+KY +
- eval(verbose=False) Tuple[ndarray, list] [source]¶
return value and policy for current state.
need to call
load_eval()
in advance
- last_to() Square | None [source]¶
the destination square of the last move or None
>>> shogi = miniosl.UI() >>> shogi.last_to() >>> _ = shogi.make_move('+2726FU') >>> shogi.last_to() == miniosl.Square(2, 6) True
- load_eval(path: str = '', device: str = '', torch_cfg: dict = {})[source]¶
load parameters of evaluation function from file
parameters will be passed to
inference.load()
.
- load_record(src: str | BaseState | MiniRecord = '')[source]¶
load a game record from various sources.
- Parameters:
src –
BaseState
orMiniRecord
or URL or filepath containing .csa or usi.
- load_record_set(path: str, idx: int)[source]¶
load idx-th game record in
RecordSet
- Parameters:
path – filepath for .npz generated by
RecordSet.save_npz()
or sfen text
- make_opening_db_from_sfen(filename: str, threshold: int = 100)[source]¶
make opening tree from sfen records, and save in npz
- mcts(budget: int = 100, report: int = 4, *, batch_size=1, resume_root=None)[source]¶
run mcts - budegt: number of simulations - report: number of (interim) report during search
- opening_moves()[source]¶
retrieve or show opening moves.
Note: need to load data by
load_opening_tree()
in advance
- pv_to_ja(pv: list[minioslcc.Move]) list[str] [source]¶
show moves in Japanese
- show_features(plane_id: int | str, *args, **kwargs)[source]¶
visualize features in matplotlib.
- Parameters:
plane_id –
'pieces'
|'hands'
|'lastmove'
|'long'
|'safety'
, or integer id (in internal representation)
- to_img(*args, **kwargs)[source]¶
show state in matplotlib image
- Returns:
an image shown in colab or jupyter notebooks
- to_np_state_feature() array [source]¶
make tensor of feature for the current state (w/o history).
44ch each 9x9 channel is responsible for a specific piece type and color - where each element is 1 (0) for existence (absent) of the piece for the first 30ch - filled by the same value indicating number of hand pieces for the latter 14ch
13ch for heuristic features
Training with torch¶
- class miniosl.StandardNetwork(*, in_channels: int, channels: int, out_channels: int, auxout_channels: int, num_blocks: int, value_head_hidden: int = 256, broadcast_every: int = 3)[source]¶
Bases:
PVNetwork
Standard residual networks with bottleneck architecture
- Parameters:
in_channels – number of channels in input features,
channels – number of channels in main body,
out_channels – number of channels in policy_head,
auxout_channels – number of channels in miscellaneous output,
value_head_hidden – hidden units in the last layer in the value head
- class miniosl.GameDataset(window_size: int, block_unit: int, batch_with_collate: bool = True)[source]¶
Bases:
Dataset
dataset for training.
sample position by game index (a random position in the game record is returned)
keep the latest GameRecordBlock s (e.g., 50) as in MuZero
- Parameters:
window_size – number of game records to keep
block_unit – number of game records for a block, that is a unit in add/replace oporation
batch_with_collate – need to specify collate_fn=lambda indices: dataset.collate(indices) for trainloader, if (and only if) True
Handle game records¶
see also Game record section, MiniRecord
and RecordSet
- miniosl.load_record_set(path: str, name: str = '', *, limit: int | None = None)[source]¶
load
miniosl.RecordSet
from npz file
Visualization¶
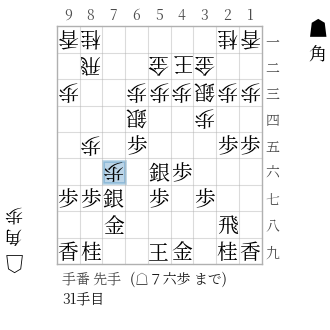
- miniosl.state_to_img(state: BaseState, *, decorate: bool = False, plane: ndarray | None = None, plane_color: str = 'orange', last_move_ja: str = '', last_to: Square | None = None, move_number: int = 0, repeat_distance: int = 0, repeat_count: int = 0, flip_if_white: bool = False, id: int = 4081) Figure [source]¶
make
ShogiFig
object including matplotlib figure as .fig- Parameters:
state – state,
decorate – highlight king location and piece covers for each color,
plane – 9x9 numpy array to make a mark on squares,
last_move_ja – last move in japanese,
last_to – the destination square of the last move,
move_number – ply in a game record,
repeat_distance – distance to the latest same position,
repeat_count – number of the occurrence of this state,
flip_if_white – rotate180() if white to move
Use external engines¶
- class miniosl.UsiProcess(path_and_args: list[str], setoptions: list[str] = ['setoption name Threads value 1', 'setoption name USI_Hash value 16'], cwd: str | None = None)[source]¶
Proxy for usi process
create process, initilaze its options and wait until
readyok
- Parameters:
path_and_args – list of path and argments
- class miniosl.UsiPlayer(engine: UsiProcess)[source]¶
bridge between usi engine and
CPUPlayer
for self-play withGameArray
.- think(self: minioslcc.SingleCPUPlayer, arg0: str) minioslcc.Move [source]¶
- class miniosl.CPUPlayer¶
adaptor for PlayerArray
- Parameters:
player – object descendant of SingleCPUPlayer
greedy – indicating greedy behavior
Note
if you give a player implemented in Python, please make sure its lifetime
- name(self: minioslcc.CPUPlayer) str ¶